Procedural Cabinet Generator
A cabinet generator that identifies spaces and dimensions with programming
Background
This side-project is used to generate a cabinet with simple steps.
Platform | Engine | Project | Time Frame |
---|---|---|---|
Windows | Unity3D | Project | 3 months |
My Tasks
In this project, I am responsible for the following tasks:
- Cabinet generator
- Design classes and provide a class diagram to junior programmers to follow
- Transform given data for generating cabinet
- Spawn cabinet parts - Undo/redo functionality.
- Design structure for data and classes
- Assets control with Unity Addressable
- Create a remote Unity Addressables project for building assets
- Integrate Mircosoft Azure Blob Storage as an Addressable hosting server - Other
- Code optimization
- Code review, peer-to-peer review
- Apply coding standard
- Project management
Study
Solution of Identifying Spaces in the Cabinet
To store valid cabinet spaces in an object-oriented world, I found that the Binary Tree data structure helps me do the trick.
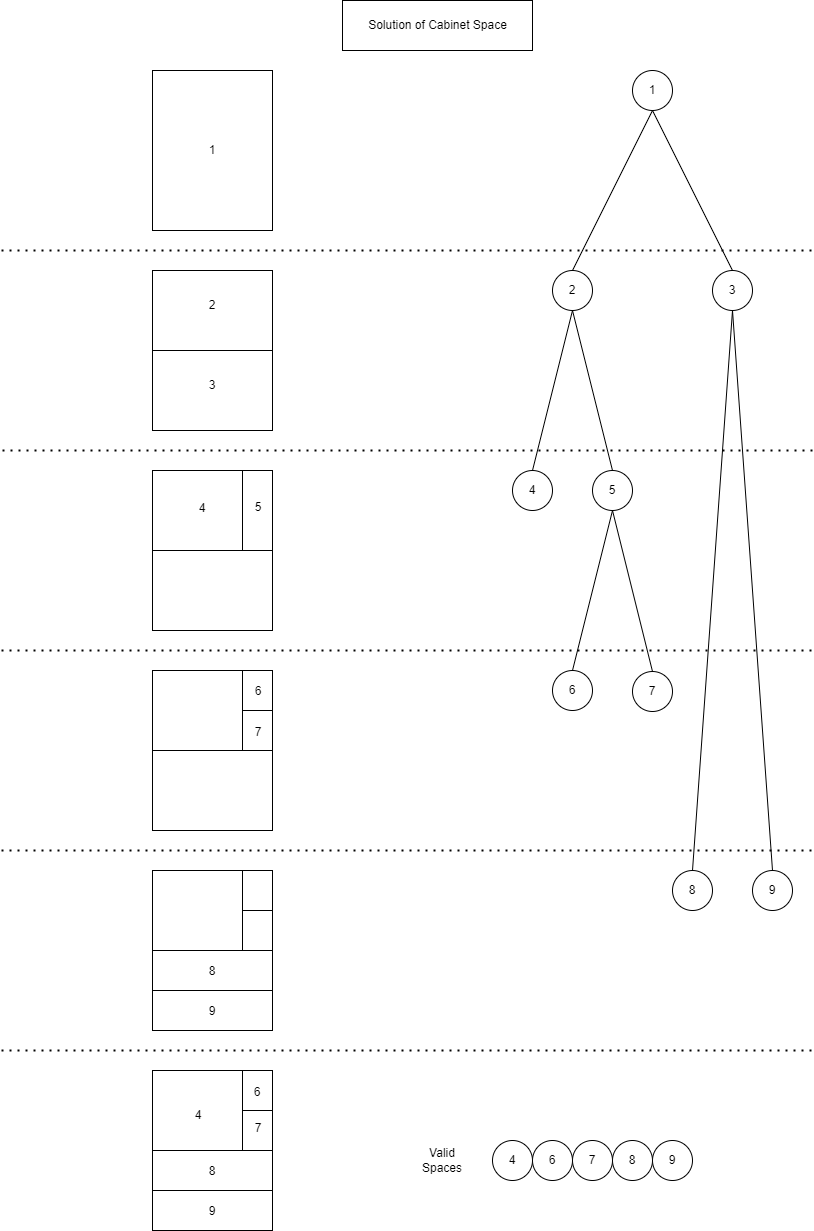
Like the figure above, each node stores left, right, and parent nodes as a reference. When the user uses a plank to separate a space, it will spawn 2 nodes (left and right or top and bottom) belonging to that node.
And we can find how many end nodes
and valid spaces we have in the cabinet space.
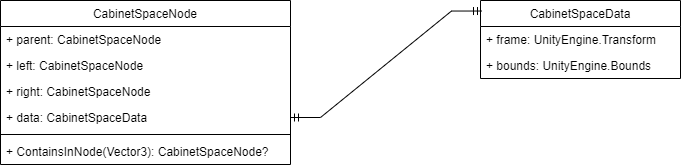
In a class diagram, I tried to design the structure like the figure. Every node will have a data
field that contains Transform
and Bounds
That helps me find which node the user will separate or place structure and item into.
Undo Redo with Command Pattern
About the undo/redo functionality, I used Command Pattern to implement the manager class that stores command, a cursor to the current command and interfaces.
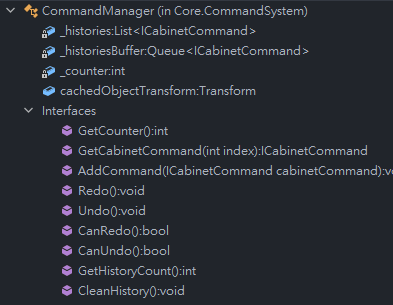
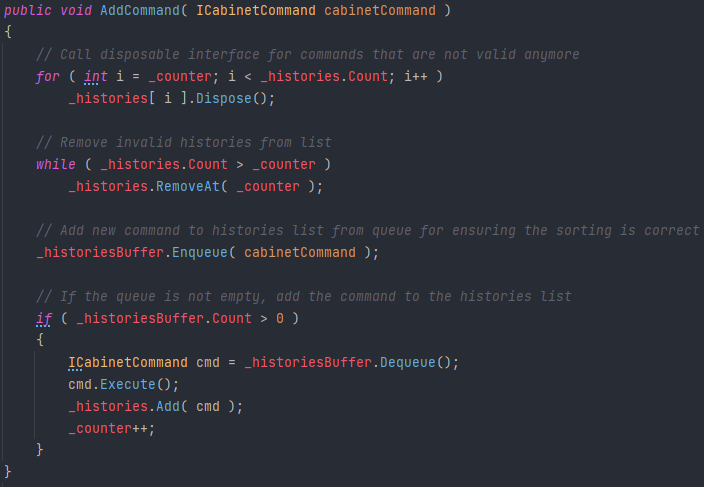
To develop a flexible and expandable environment, I created an interface - ICabinetCommand
which allows the computer to enforce specific properties on a class.

As a result, the command manager will only call Execute
and Undo
at certain times. In the future, if some new commands need to be created, then the command manager will stay in no changes as much as possible.
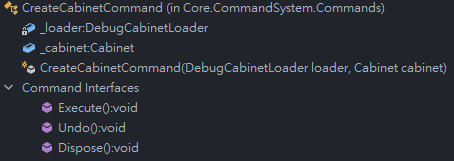
Each command will be implemented ICabinetCommand
and has its constructor. All dependencies necessary for running this user action should be passed through the constructor.
Technologies Used
- Unity3D
- Rider
- C#
- Microsoft Azure Blob Storage
Videos
First Prototype
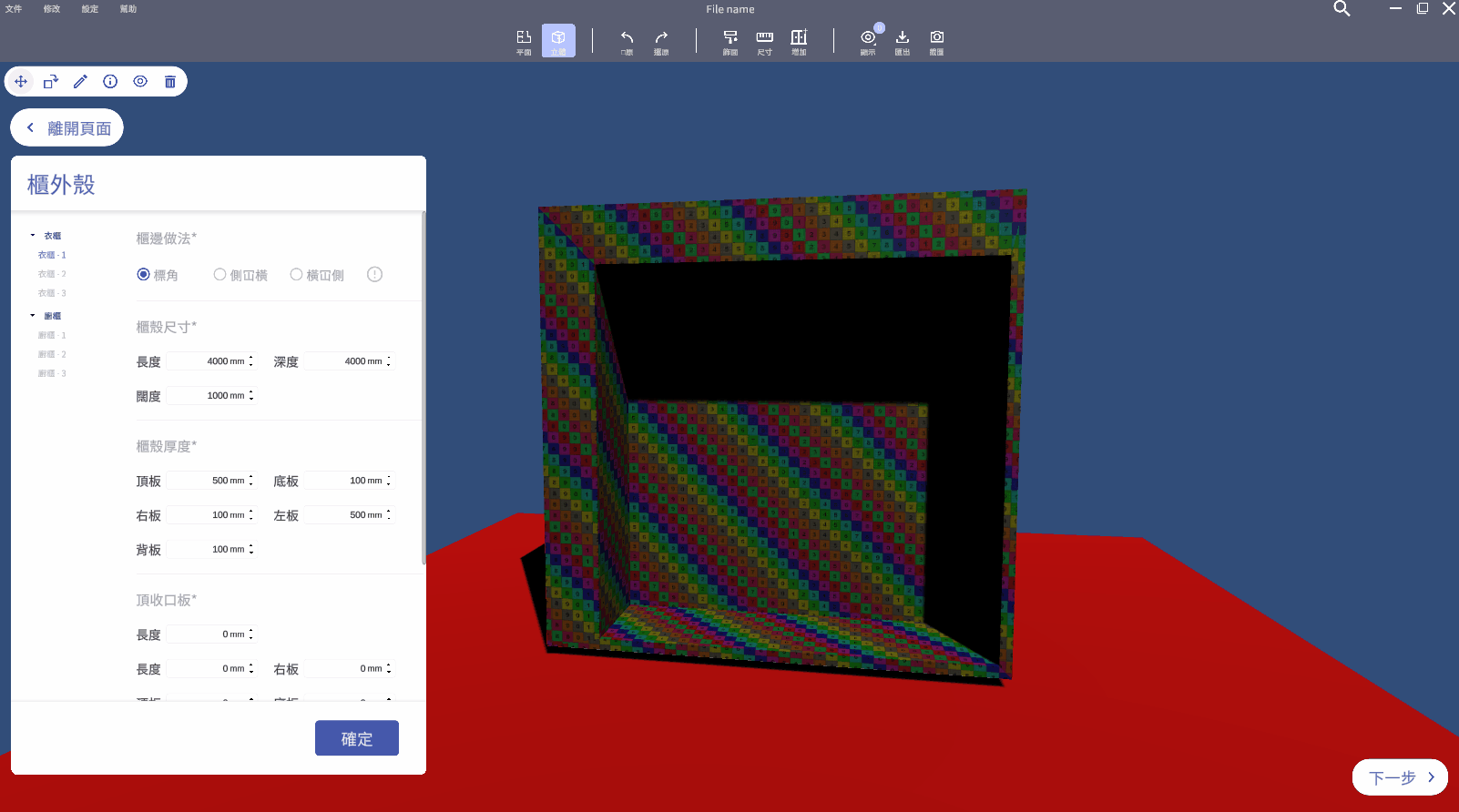
Second Prototype